The React, Angular, WebAPI, Chart FAQ
井民全, Jing, mqjing@gmail.com
The Public FAQ Index (view)
Table of contents
1. React 3
1.1. Setup 3
1.2. Debug 5
1.3. Component 7
1.3.1. OLD/BAD Practice 9
1.4. TSX/JSX 11
1.5. Test 14
1.6. UI 14
1.6.1. Layout 14
1.6.2. CSS Style 15
1.6.3. Canvas 16
1.6.4. Heading 16
1.6.5. Input 17
1.6.6. Slides 17
1.6.7. Button 17
1.6.8. Switch 19
1.6.9. Progress 19
1.6.10. Table 19
1.6.11. Form 20
1.6.12. Dialog 21
1.6.13. Calendar 21
1.6.14. Menu 21
1.6.15. Tree View 22
1.7. Server-related 24
1.8. CRUD 24
1.9. Redux 25
1.10. Auth: for User Signup & User Login 27
2. Angular 27
2.1. Common 27
2.2. App 27
2.3. Component 28
2.4. CRUD 29
2.5. Debug 29
3. HTML5 30
3.1. List item 31
3.2. File 32
3.3. Button 33
3.4. Request 36
4. CSS 37
5. UI Framework 38
6. Authortication 39
6.1. Common 39
7. WebAPI 40
7.1. Console 40
7.2. Keyboard 41
7.3. Touch 41
7.4. Timer 41
7.5. WebBluetooth 42
7.6. WebAudio 43
7.7. WebRTC 46
7.8. WebAssembly 47
7.9. WebSerial 47
8. Animation libraries 47
8.1. Spring 47
8.2. Famer 48
9. Graphic libraries 48
9.1. Survey 48
9.2. 3D libraries 48
9.3. Image libraries 48
9.4. Map, CesiumJS 48
10. Chart libraries 49
10.1. comm 49
10.2. High performance chart libraries 49
10.2.1. Dygraphs 49
10.2.2. TimeChart 50
10.2.3. uPlot 51
10.2.4. Webgl-plot 51
10.2.5. [canvas] d3 52
10.2.6. [canvas] Wavesurfer 52
10.2.7. Livechart 52
10.2.8. Chart.js 53
10.2.8.1. Javascript (chart.js only) 53
10.2.8.2. Typescript + React 53
10.2.8.3. react-chartjs-2 54
10.2.8.4. chartjs-plug-stream 54
10.2.9. Recharts 57
10.2.10. Others 57
1. React
[react, example] React.js Examples (ref)
1.1. Setup
[react, sheet] The React sheet (sheet)
[react, official] React (ref), State (ref)
[react, official, tutorial] React (ref)
[react, doc, api] api doc (ref)
[react, official, example] The official live example (ref)
[react, install] How to install react via npm (ref)l
npm init npm install --save react npm install --save react-dom |
[react, port] How to setup the default port for React app (ref)
# package.json (Ubuntu) "scripts": { "start": "PORT=8000 react-scripts start", "build": "react-scripts build", "test": "react-scripts test", "eject": "react-scripts eject" },
|
[react, app, typescript] Create typescript react app (ref) (blog)
npx create-react-app my-app --template typescript cd my-app npm start
|
[react, app, javascript] Create react app (ref)
npx create-react-app my-app |
[react, app, build] release build
[react, app, env] How to pass parameters to the react app (view) (ref)
package.json "scripts": { "start:test": "REACT_APP_MY_SWITCH=TRUE react-scripts start", "start": "react-scripts start", ... } |
App.tsx const { REACT_APP_MY_SWITCH } = process.env |
yarn test Output REACT_APP_MY_SWITCH = undefined |
[yarn, script, makeclean, cross-platform] A npm script for clean
Install yarn global add rimraf // yarn add rimraf -D
clean "clean": "rimraf out build dist node_modules & rimraf **/*.js & rimraf **/*.map & rimraf **/*.raw & rimraf **/*.log", |
[react, makeclean] How to clean the React App
// package.json "clean": "rm -fr node_modules" |
1.2. Debug
[react, debug, basic] How to add a break point in code
xxx{){ ... debugger; ... } |
[react, debug, tool] Debug tool for firefox (ref)
[react, debug, tool] Debug tool for Chrome (ref)
Step 1: Chrome web store -> React Developer Tools 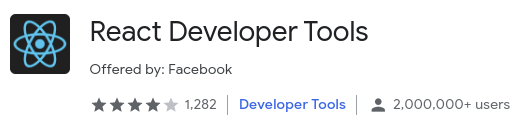
Step 2: Mouse right click to bring up the inspect tool
Step 3: Check the componset tree in [⚛️ Components] tabs 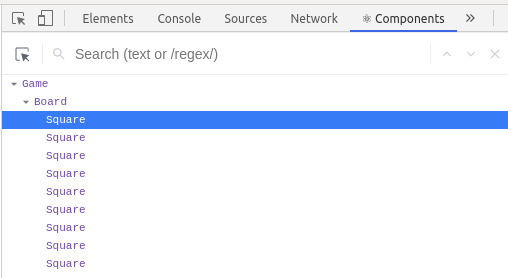
|
[react, debug, vscode] How to config the chrome debugger in vscode (ref)
Step 1: Create a launch.json file that chooses chrome Step 2: Change the port
{ "version": "0.2.0", "configurations": [ { "type": "chrome", "request": "launch", "name": "Launch Chrome against localhost", "url": "http://localhost:3000", "webRoot": "${workspaceFolder}" } ] }
|
Step 3: Build & Run service yarn start
Step 4: Click Run from vscode
|
1.3. Component
[react, function component] 更新元件狀態不使用 Ref 的範例 Create a function component update state without Ref (github) (modify to setState(state => ({...state, id: 123}));)
[react, function component] 更新狀態是直接呼叫 function 給參數, 回傳 JSX 顯示畫面
元件狀態模板: const [state, setState] = useState({ id: 0 });
更新狀態模板: setState(... ); // 會啟動連鎖變更繪圖 (ref)
// function component setState(state => ({...state, id: 123}));
// bad setState({...state, id: 123}); // this is the class component update form |
Render 元件設定 props 模板: <MyFunComp id={state.id}>
元件模板
function MyFunComp(props:{id:number}): JSX.Element { if(pros.id == 0){ return (<div> GOT 0</div>); }
return(<div> Others</div>); }
export default MyFunComp; |
[react, function component, create] How to create a React Component using function***(view)
一個簡單的好例子 (ref)
不要在 function component 裡面用 ref (ref2)
不要過度使用 ref 改變 component 狀態, 而使用 props 和 setState 產生連鎖反應改變元件狀態
[react, function component, instance variable] How to use non-state variables in functional components (ref1), (ref2)
const bcheckedRef:any = useRef<boolean>(); // 宣告 bcheckedRef.current = true; // write console.log(bcheckedRef.current); // read |
[react, function component, effect] How to run only once (ref)
useEffect( () => { RunOnlyOnce(); }, []);
|
[react, function componen, hook, useCallback] 如何使用 useCallback 更新 React function component 的狀態 (view) (blog), 被動的 update. useEffect 是在 render 後, 被呼叫.. 而 setState 則是主動更新.
[react, class component, prop, pass data] How to pass data between Components via props (view) (ref)
[react, class component, prop, pass function] How to pass props to the Class Component (view)
[react, class component, state] How to use this.state in Class component (view)
type MyState = { date: Date }; // define the state data type class App extends React.Component<{}, MyState> { ... } |
[react, class component, state] How to update the component state from javascript to the html render via setStatus (view) (ref) (ref2)
When you call setState in a component, React automatically updates the child components inside of it too. (ref) |
Key: // 1. 宣告 State 成員 // this.state = {變數 1: 資料1 或陣列, // 變數 2: 資料 2, // }; //
// 2. Update 資料: // this.setState({ // 變數 1: 新資料 1, // 變數 2: 新資料 2, });
Detail import React from 'react'; class A extends Reacts.Component { constructor(props) { super(props); this.state = {Count : 0}; // Initial component state
this.onClicked = this.onClicked.bind(this); }
onClicked () { let local_count = this.state.Count + 1; this.setState({Count: local_count}); // Update the state }
render() { return ( <div className="A"> abc = <span> {this.state.Count} </span> <button onClick={this.onMyClick}> Click</button> </div> ); } } |
1.3.1. OLD/BAD Pratice
[component, lifecycle] The react component lifecycle (ref)
// Class component (full text) export class xxxView extends React.Component { constructor(props) componentDidMount() componentWillUnmount() render() } |
// Functional component export const xxxView = () => { const xxxDomRef = useRef(); // create connect useEffect( () => { // access xxxDomRef.current return () => { subscription.unsubscribe(); }; }, [props.source]);
return <div ref={xxxDomRef} />; } |
[react, class component, create] How to create a React component using class**** (view), (github)
[react, class component, access] How to access the React component
盡量少用 Ref, 應該使用 props 搭配 setState 產生連鎖變更狀
Ref (ref) querySelector (ref) let canvas = document.querySelector('canvas');
|
[react, class component, image] How to switch the image when user clicked the button (view), (blog),
使用 Ref 來更新元件是不恰當的
[react, class component, image] How to add static asset to the React component (view), (blog)
盡量少用 class component, function component 非常優雅
[react, class component, state] How to use map to handle multiple state change in React component (view)
盡量少用其他奇奇怪怪的資料型態, 應該直接使用 type object 來宣告元件的 state
[react, componentDidMount]
在 function component 中, 對應的功能是 effect
[react, ref, callback] How to use callback format to the Ref (ref)
盡量少用 Ref
1.4. TSX/JSX
[tsx, vscode] Show Error when opening TSX file: Cannot use JSX unless '--jsx' flag is provided ? (ref)
Step 1: Open tsconfig.json Step 2: Setup jsx: flag From "jsx": "react-jsx" to "jsx": "react" |
[tsx, html] JSX vs. HTML (ref)
// HTML (ref): Almost elements require opening and closing tags <a href="https://www.w3schools.com">Visit W3Schools.com!</a>
// JSX: any element can be writed as self-closing tag const element = <a href={user.avatarUrl} />; |
JSX: JSX is JavaScript XML, an extension of JavaScript that allows writing HTML in React. HTML: a standard markup language for documents designed for the web browser
|
[react, render] How react renders a string from jsx (ref)
[jsx] jsx compile (javascript) (view)
<component props=...> children </component>
=> React.createElement(component, props, ...children) |
[jsx, brace] What's the differente betwee double brace and single brace for inline styles
Ex: function(text, index) { return ( <div style={{textDescription: 'hello'}} className="todo"> {text} </div>; ); }
{text} : In JSX {variable}, the braces are used to reference the JS variable or expression. {{textDescription: 'hello'}} : the outer braces denotes a js expression and the inner braces are for creating a object.
|
[tsx, create] How to return a JSX.Element?
class App ... {
private __arrayTag:any = [];
constructor(){ this.addTag(); }
addTag(){ this.__arrayTag.push(<p> item </p>) }
render() { return ( <div> <p>Hello World</p> {this.__arrayTag} </div> ); }
} |
[tsx, show, array] How to show array data (ref)
import React from "react"; import axios from "axios";
export default class App extends React.Component { state = { users: [], }; componentDidMount() { axios.get("/users.json").then((response) => { this.setState({ users: response.data }); }); }
render() { const { users } = this.state; return ( <div> <ul className="users"> {users.map((user) => ( <li className="user"> <p> <strong>Name:</strong> {user.name} </p> </li> ))} </ul> </div> ); } } |
1.5. Test
[test, survey] 15 Best Chrome Extensions for Software Testers in 2021 (ref)
[react, test, official] The React Test Overview (ref)
1.6. UI
[react, ui, doc] React components for efficiently rendering large lists and tabular data -- react-virtualized
[react, ui, survey] 20+ Best React UI Component Libraries / Frameworks (ref 1) (ref 2)
[react, semantic] Semantic-UI-React (github)
[react, bootstrap] Frontend framework, Bootstrap (ref)
[react, ui, material] Material Kit React (github), (doc), (ref)
[ract, ui, material] Color (ref)
1.6.1. Layout
[react, material-ui, layout, grid] How to use grid layout (ref)
[react, material-ui, layout, grid] How to get the width of cell (ref)
1.6.2. CSS Style
[css, tailwindcss] Rapidly build modern websites without ever leaving your HTML (ref)
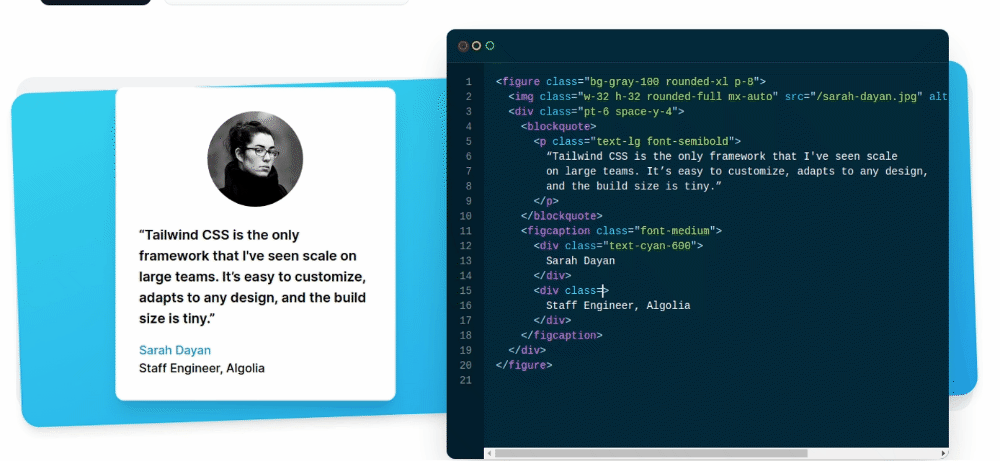
[react, render, css, inline] How to setup the style in jsx (ref)
class App extends Component { graphStyle = { width: '1500px', height: '300px', };
// ...
render() { return ( <div> <div id="graph" style={this.graphStyle}></div> </div> ); } }
|
[react, css, file] How to use css in file (ref)
// File: xxx.tsx import React from 'react'; import './DottedBox.css';
const DottedBox = () => ( <div className="DottedBox"> <p className="DottedBox_content">Get started with CSS styling</p> </div> );
export default DottedBox; |
/* File: DottedBox.css */ .DottedBox { margin: 40px; border: 5px dotted pink; }
.DottedBox_content { font-size: 15px; text-align: center; }
|
1.6.3. Canvas
[react, canvas] How to create a canvas using react (view)
export default class App extends React.Component { ...
render() { return ( <div className="App" > <div className="canvasWave"><canvas width="600" height="300"></canvas></div> </div> ); } } |
1.6.4. Heading
[react, h2] How to write a hello world react app (view)
1.6.5. Input
[react, input, example] 連動計算的範例 (view) (demo)
[react, checkbox] An example for the checkbox (view)
[react, form, submit] How to handle submit (view)
1.6.6. Slides
[react, slide] How to get the HTML slide object? (view)
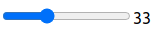
1.6.7. Button
[react, button, animated] 3D animated react button (ref) (ref2) xxx
[react, material-ui, button] How to use material-ui button in React app (view)
yarn add @material-ui/core
# code import Button from '@material-ui/core/Button'; ... <Button onClick={onClickHandler} variant="contained" color="primary"> {state} </Button> |
[react, button, callback] How to handle the callback function (view)
[react, material-ui, button] Title always upper case (ref)
[react, button] How to add onClick event to a button component (view)
// key: // Step 1: UI part: find the render method // Step 2: add the html onClick event // ref class Square extends React.Component { render() { return ( <button className='square' onClick={function() {alert('click');}}> { this.props.value } </button> ); } }
|
import React from 'react'; export default class App extends React.Component { constructor(props:any) { super(props); this.onClicked = this.onClicked.bind(this); }
onClicked () { console.log('click'); }
render() { return ( <div className="App"> <button onClick={this.onClicked}> Play</button> </div> ); } }
|
[react, button, evergreen] How to create a evergreen button using react (ref)
npx create-react-app my-app cd my-app yarn add evergreen-ui
// File: ./src/App.js import { Button } from 'evergreen-ui'
<div className="App"> .... <Button appearance="primary">I am using 🌲 Evergreen!</Button> </div> |
|
1.6.8. Switch
[react, material-ui, switch] How to use Material-ui Switch component (view)
1.6.9. Progress
[react, material-ui, progress] The progress (ref)
1.6.10. Table
[react, spreadsheet] How to createa spreadsheet using react (ref)
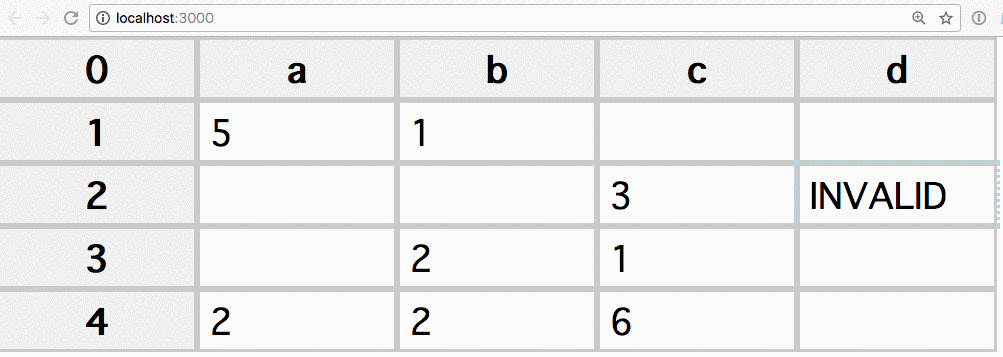
[react, spreadsheet] React component like SpreadSheet (ref)
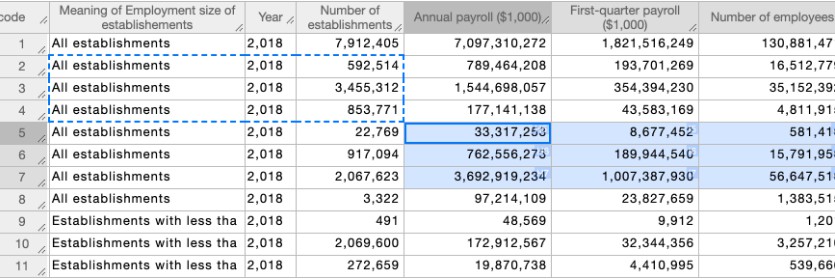
[react, spreadsheet, google] How to access and display googl sheets using reactorjs (ref)
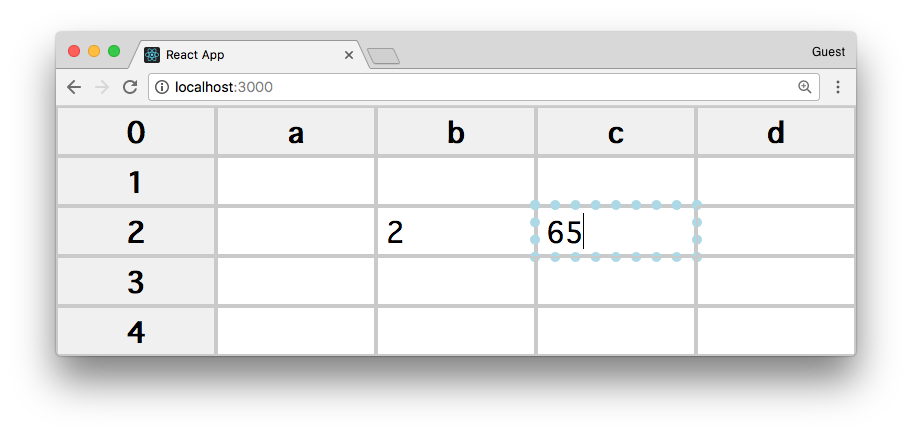
[react, grid] How to create a spreadsheet -- adgrid (ref)
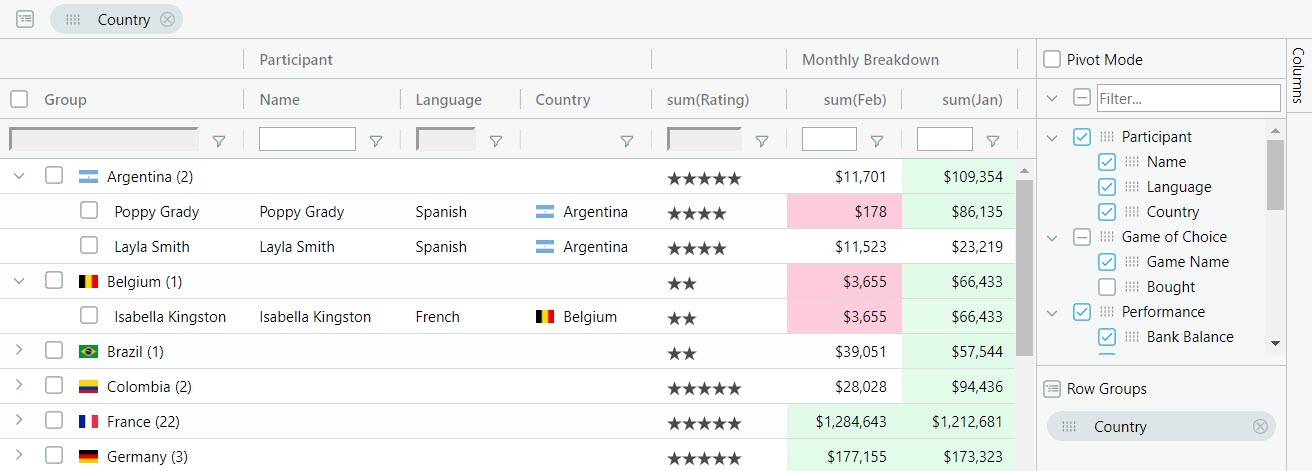
[react, table, crud] React Table CRUD example using Hooks & react-table v7 (ref)
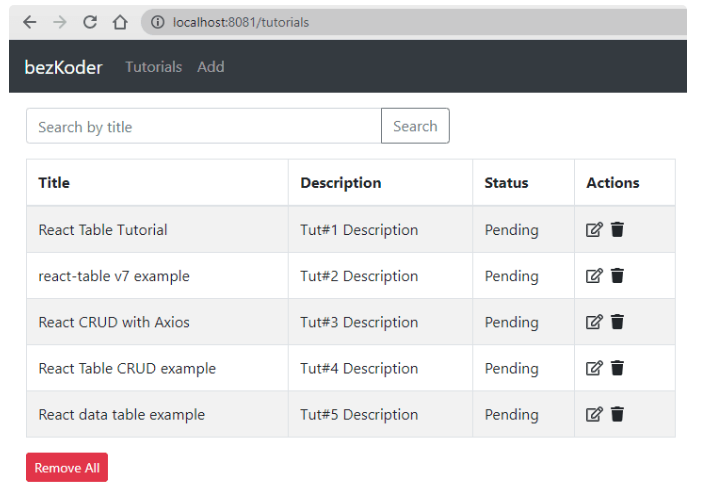
[react, table, crud] React Hooks CRUD example with Axios and Web API (ref)
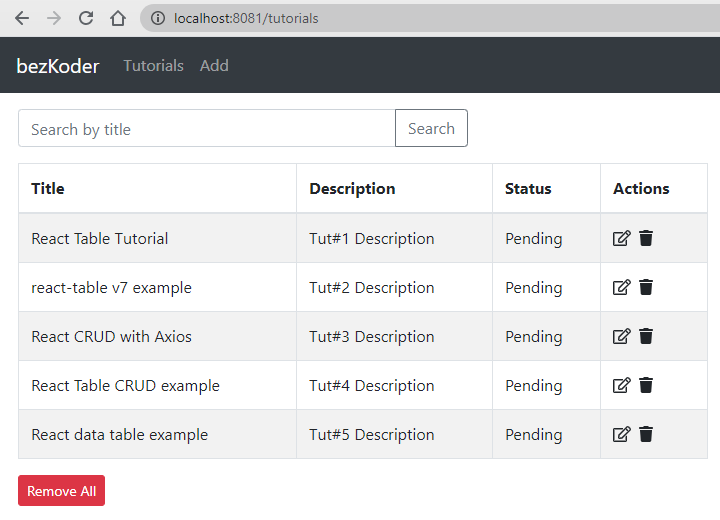
1.6.11. Form
[react, ui, form] formik (ref)
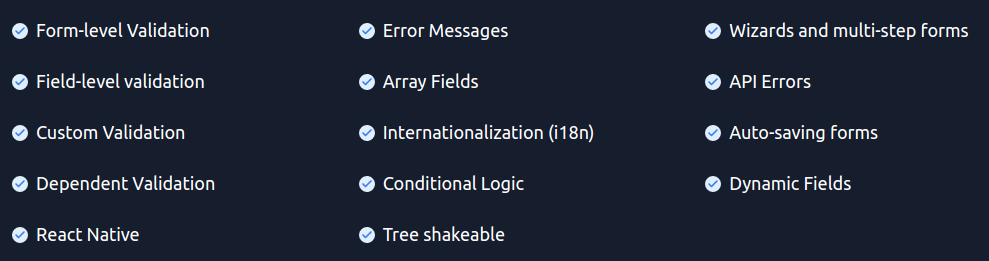
1.6.12. Dialog
[react, ui, dialog] How to create a dialog using React SkyLight (ref)
1.6.13. Calendar
[react, ui, calendar] A Survey on Calendar (view)
1.6.14. Menu
[react, ui, menu] Examples (view)
[react, ui, menu] A ReactJS circular menu (ref)
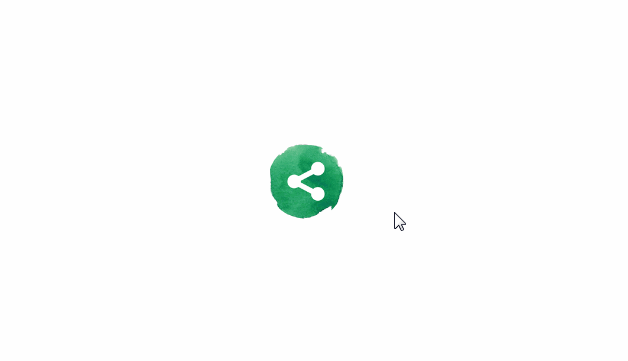
[react, ui, menu] A stateless tree menu component for React (ref)
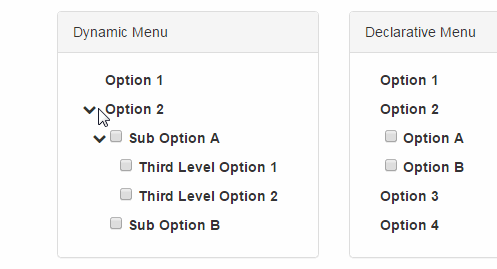
[react, ui, menu] Path Fly Out menu recreated using React Motion (ref)
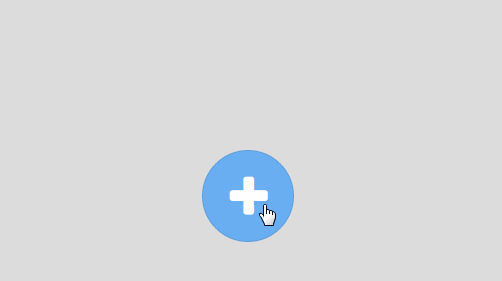
1.6.15. Tree View
[react, material, tree] The material tree view (ref)
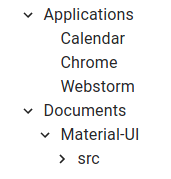
[react, tree, draggable] A Draggable tree component for react (ref)
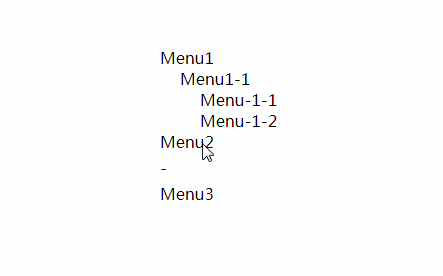
[react, tree, draggable] react-sortable-tree (ref), (ref_survey)
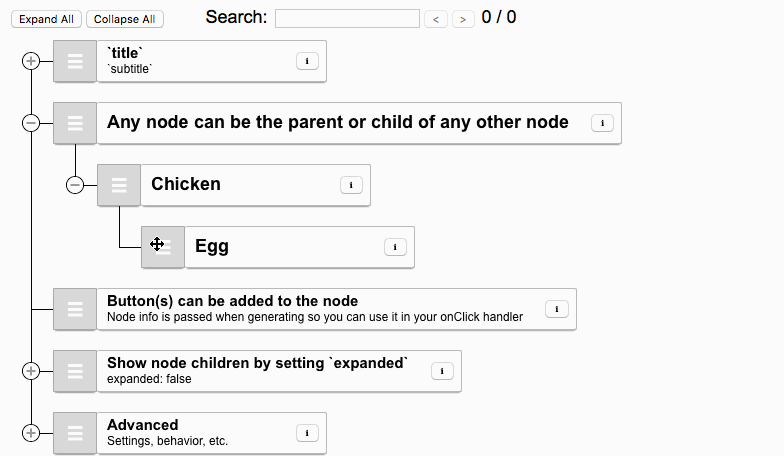
[react, tree, search] TreeView with Search Bar (ref)
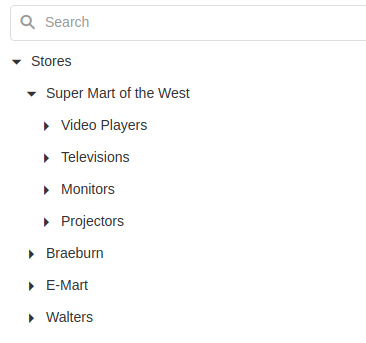
[react, tree] deni-react-treeview (ref), (ref_survey)
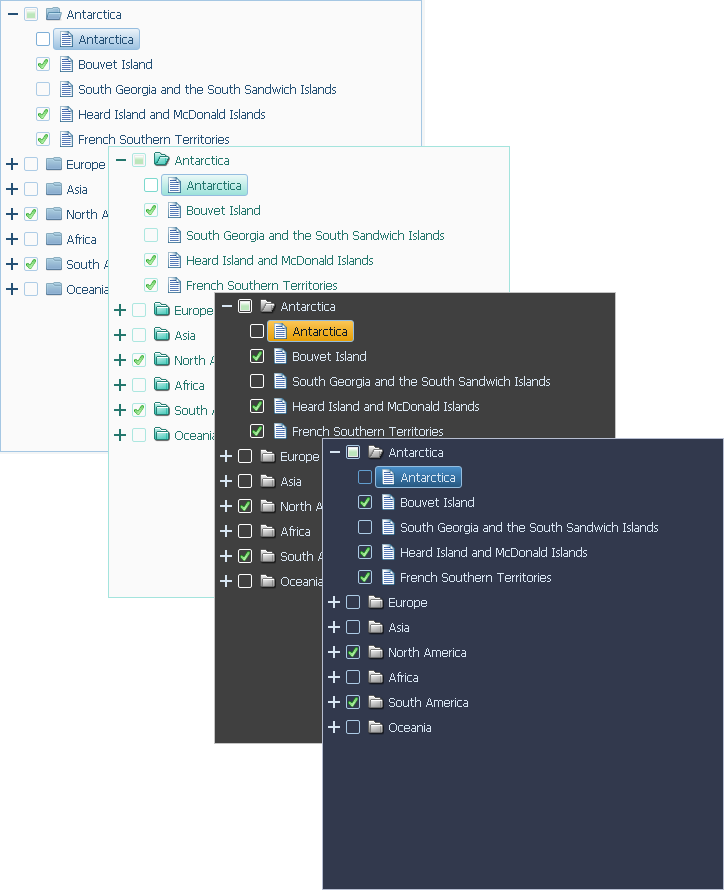
[react, tree] Treebeard (ref), (ref_survey)
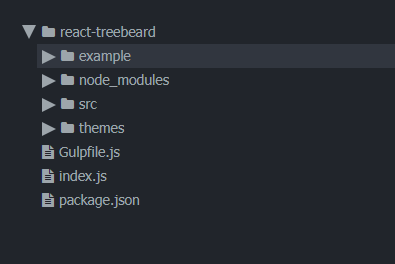
[react, tree, animation] The animation tree (ref), (ref_survey)
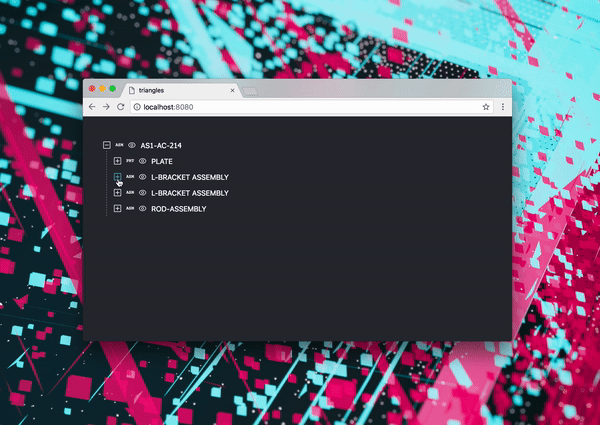
1.7. Server-related
[react, flask] React and Flask Full Stack Web app: Component-oriented and Data-driven (ref)
[react, express, deploy] How to deploy the React App with Express (view)
[react, express, restfulAPI] How to implement a restfulAPI using Express and React (view)
[react, express, sse] How to create server that streams events to the front-end client using Express and React**** (view), (blog)
[react, express, sse, get] How to create a express server accepting RestfulAPI to change it's status showing on client (view), draft
[heroku, react] Deploy your React app on Heroku (view)
1.8. CRUD
[react-admin] react-admin (sheet), (ref)
[react-admin] Bootstrap the react-admin (view), (blog)
[react-admin, firebase] How to use react-admin with Firebase (draft)
[react, admin] CRM Starter Admin App built with react and Ant Design (ref)
[react, framework, admin] reduction-admin/react-reduction (github), (demo)
[react, framework, admin] Notus React FREE TAILWIND CSS UI KIT AND ADMIN (ref), (angular)
[react, flask, crud] Build a Simple CRUD (Create, read, update and delete) App with Python, Flask, and React (ref)
1.9. Redux
[redux, official] The official site (ref)
a library for managing and updating application state, using events called "actions"
[redux, debug] The Redux DevTools (ref)
[redux, flow] What the data flow looks like?
Store: 統一從 App Store 讀取 State
Action (Event Handler): 透過 Dispatch 更改 App Store 中的 state
Reducer: 定義 update logic, 下一個 state, 根據目前 state 以及 action.
[redux, action] What is the action in redux?
An action is an event that descripts something that happened in the application. [ref] |
// A typical action object const addTodoAction = { type: 'todos/todoAdded', // type: category/eventName payload: 'Buy milk' // additional information }
|
[redux, action] What is the action creator in redux?
A action creator is a function used to create an action object |
// A typical action creator function const addTodo = text => { return { type: 'todos/todoAdded', payload: text } }
|
[redux, reducer] What is the reducer in redux?
A function that decides how to update the state based on the current statue and an action object. |
// A typical reducer function const initialState = { value: 0 }
function counterReducer(state = initialState, action) { if (action.type === 'counter/increment') { // 回傳新的 state 物件 return { ...state, // 複製原來的 state value: state.value + 1 // Update state.value } } return state }
|
[redux, store] What is the store in redux?
The current Redux application state lives in the store. |
// Create a store import { configureStore } from '@reduxjs/toolkit'
const store = configureStore({ reducer: counterReducer })
console.log(store.getState()) // {value: 0}
|
[redux, dispatch] What is the dispatch?
The only way to update the application state method is call the store.dispatch(action). The store will save the updated state in side. |
[redux, thunk, official] The official site of redux-thunk (ref)
[react, redux] How create a React + Redux App (view), (blog)
1.10. Auth: for User Signup & User Login
[auth, main] Auth: for User Login (view)
2. Angular
2.1. Common
[angular, sheet] The Angular sheet (view)
[official, doc] Google Angular (ref)
[angular cli, version] How to check the Angular CLI version (ref)
[angular, cli, offical] The Angular cli official site (ref)
[angular cli, version] How to downgrade the Angular CLI version (ref)(ref2-typescript)
sudo npm uninstall -g @angular/cli sudo npm cache clean sudo npm install -g @angular/cli@1.4.1 |
[angular, tslint, issue] Not using the local TSLint version found for xxx To enable code execution from the current workspace you must enable workspace library execution.(ref)
2.2. App
[angular, app] Creating a single page todo app with node and angular (ref)
[angular, app] How to create an Angular application in one step (view)
Step 1: Create template app ng new angular-tour-of-heroes cd angular-tour-of-heroes yarn start # ng serve --open
Step 2: Modify the template File: src/app/app.component.html
|
[angular, style] How to setup a consistent look across all components in application? (ref)
2.3. Component
[angular, component, create] How to create a angular component
Command ng generate component my-comp
Change Create: Define the new component folder: app/my-comp files: my-comp/my-comp.component.ts
...selector: 'app-my-comp', // <<--- the element selector |
my-comp/my-comp.component.html
Update files: app.module.ts
|
[angular, component, show] How to display the component in App component?
Key // Add the the element selector to the app.component html template ex: <app-heroes></app-heroes> |
[angular, component, status, update] How to update the Component status (view)
Key: export class xxxComponent{ // <-- component.ts variable = 'xxxx' }
{{variable}} // <---- html
Detail // File: app.component.ts ... export class AppComponent { title = 'Tour of Heroes'; }
// File: app.component.html <h1>{{title}}</h1> |
[angular, component, button] How to create a button using Angular in 3 steps (view)
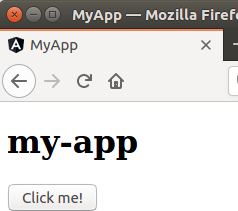
2.4. CRUD
[react, framework, admin] Notus Angular FREE TAILWIND CSS UI KIT AND ADMIN (react), (angular)
2.5. Debug
[alert] How to show a message-box liked window
window.alert("sometext");
3. HTML5
[html, color] color (ref), (color map)
[html, css, display] How to specify an html element is display (view)
Key: block: 佔據整行 In-line: 全部放在一行 none: 不顯示
Code: <style> li { display: block; } </style> |
[html, code] URL decoder (ref)
%E9%87%91%E8%8F%AF92 --> 華山92 |
[html, sumit, get] How to create a GET form (ref)
[html, alert] How to show alert box (ref)
alert("I am an alert box!"); |
[html, document] How to write text on the page
Key: document.write(i.toString() + '<br>');
Example: for(let i = 1; i<7; i++){ document.write(i.toString() + '<br>'); } |
[html, mine] The Media Type (ref), all availbe Media type (ref)
[html, javascript] How to embed javascript code to html file (view)
<body> <script> </script> </body> |
[html, javascript] How to embed javascript file to html (ref)
<script src="myscripts.js"></script> |
[html, document, getElementById] How to query a html element by id (view), (blog)
[html, canvas, data] How to convert the HTML canvas to dataURL (ref)
var canvas = document.getElementById('canvas'); var dataURL = canvas.toDataURL(); console.log(dataURL); // "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNby // blAAAADElEQVQImWNgoBMAAABpAAFEI8ARAAAAAElFTkSuQmCC"
|
[html, dataURL, blob ] How to convert the dataURL to binary blob (view)
3.1. List item
[html, item] The normal form (ref)
<ul> <li>Coffee</li> <li>Tea</li> <li>Milk</li> </ul>
|
[html, item, js, list2item] How to create a item list from a list (ref)
const candies = ['snickers', 'skittles', 'twix', 'milky way'] <ul> {candies.map(candy => ( <li key={candy}> <button onClick={() => dispense(candy)}>grab</button> {candy} </li> ))} </ul>
|
3.2. File
[html, file] How to select a file from browser (ref)
public browserSelectFile(){ var input = document.createElement('input'); input.type = 'file'; input.addEventListener('change', (event) => { if(event.target === null){ LoggerService.logger.warning('[Warn] selectFileBrowser, event.target === null. (User cancel?). Act: do nothing.'); return; } const fileList = (event.target as HTMLInputElement).files; console.log(fileList); });
input.click();
} |
[html, file] Read the content from file (ref)
var input = document.createElement('input'); input.type = 'file';
input.onchange = e => {
// getting a hold of the file reference var file = e.target.files[0];
// setting up the reader var reader = new FileReader(); reader.readAsText(file,'UTF-8');
// here we tell the reader what to do when it's done reading... reader.onload = readerEvent => { var content = readerEvent.target.result; // this is the content! console.log( content ); }
}
input.click();
|
[html, file, binary] How to read binary file
3.3. Button
[html, button ,create] How to create a button with ID
let button = document.createElement('button'); button.setAttribute("id", strID); |
[html, button, create] How to create a button (view)
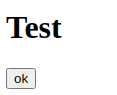
<html lang="en"> <body> <script type="application/javascript"> let button = document.createElement('button'); button.innerHTML = 'ok'; // set the button content = "ok" button.addEventListener('click', function() { console.log('clicked'); }); var body = document.getElementsByTagName("body")[0]; body.appendChild(button); </script> </html>
|
[html, button, get] How to get the button element by Id
let btPlay = document.getElementById("btPlayId"); if(btPlay === null){ console.log('[Error] btPlay === null'); return; } btPlay.innerText = 'playing'; |
[html, button, text] How to change the html button text?
let btPlay = document.getElementById("btPlayId"); if(btPlay === null){ console.log('[Error] btPlay === null'); return; } btPlay.innerText = 'playing'; |
[html, button, addEventListener] How to add an EventListener (ref)
Use => allow function
class MyClass{ initUI(){ let button = document.createElement('button'); button.innerHTML = 'ok'; const that = this; button.addEventListener('click', => () { // this.constructor.name => MyClass console.log('this.constructor.name = ', this.constructor.name); this.myFun(); }); } myFun(){ console.log('myFun()'); } } // end of class |
Use a const that to save this reference
class MyClass{ initUI(){ let button = document.createElement('button'); button.innerHTML = 'ok'; const that = this; button.addEventListener('click', function () { // this => HTMLButtonElement console.log('this.constructor.name = ', this.constructor.name);
// that => MyClass console.log('that.constructor.name = ', that.constructor.name); that.myFun(); } ); } myFun(){ console.log('myFun()'); } } // end of class |
Bind a inline anonymous function (ref)
class MyClass{ initUI(){ let button = document.createElement('button'); button.innerHTML = 'ok'; const that = this; button.addEventListener('click', function () { // this.constructor.name => MyClass console.log('this.constructor.name = ', this.constructor.name); this.myFun(); }.bind(this) ); } myFun(){ console.log('myFun()'); } } // end of class |
class MyClass{ initUI(){ let button = document.createElement('button'); button.innerHTML = 'ok'; button.addEventListener('click', evt => this.onClick(evt)); } onClick(evt){ console.log('clicked'); this.myFun(); } myFun(){ console.log('myFun()'); } } // end of class |
[html, image]
3.4. Request
[html, xhr, get] How to use GET method to access remote api with XMLHttpRequest (view)
[html, xhr, get] How to use GET method to access remote api using XMLHttpRequest with React client and Express server*** (view), (blog)
[html, xhr, get, onLoad] Use arrow function to handle onLoad event with XMLHttpRequest (ref)
class MyClass { loadAudioBuffer(url){ var request = new XMLHttpRequest(); request.open('GET', url, true); request.responseType = 'arraybuffer'; // Decode asynchronously request.onload = () => { this.context.decodeAudioData(request.response, function(theBuffer) { console.log('Get the data.') let buffer = theBuffer; console.log('buffer = ', buffer); }, function () { console.log('[Error] loadAudioBuffer:: decodeAudioData error.') }); } request.send(); } } |
[html, xhr, post, ArrayBuffer] How to post an ArrayBuffer data to server using XMLHttpRequest, React and Express *** (view), (blog)
[html, xhr, post, palaintext] How to post an plaintext data to server using XMLHttpRequest, React and Express *** (view), (blog)
[html, xhr, post, json] How to post JSON data to server using XMLHttpRequest, React, Express and AJV (view), (blog)
4. CSS
[css, tailwindcss] The Tailwindcss (ref)
[css, center] Center
.center { margin: auto; /* To horizontally center a block element, use margin:auto*/ background: lime; width: 66%; }
// Usage <div> <div>{props.labName}</div> <div id="graph" className="center"> </div> </div>
|
5. UI Framework
[ui] perfect-scrollbar (ref)
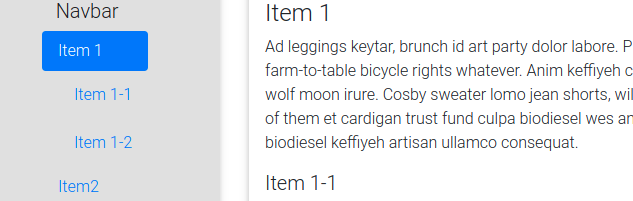
[ui] smooth scrollbar (ref)
container / +--------+ ##################### # | | # # | | # # +--------+ # -- content # # # # #####################
|
[ui, kanban] jkanban (ref) (ref-ionic)
add item, add board, delete board
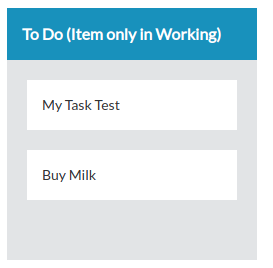
[ui, drag] dragula (ref)
[ui, booking] gantt-schedule-timeline-calendar (ref)
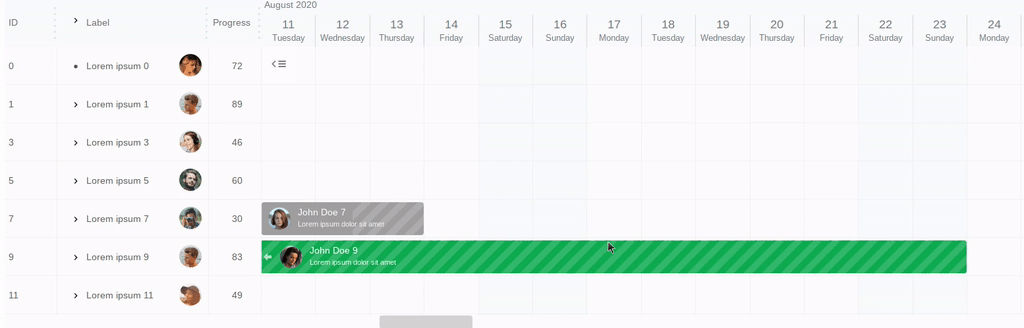
6. Authorization
[author, library, login] How to use otaka to do authorization (ref) (usage)
6.1. Common
[framework] DoJo (go)
[ui] ONSen (ref)
[ui + cordova + IDE + phone debug tool] Ionic -- IDE + ionic view (phone debug platform) + , (ref)
[bundle, cordova] Cordova = typescript + AngularJS + Onsen UI (view) (ref)
[chrome, context] chrome context menu programming (view)
[dictionary] CDICT, http://cdict.net/?q=Test
[google, sheets, json] Get the Google sheets data via json format (ref)
[google, sheets, ajax] Edit, Insert, Delete Google sheet (ref)
[Server] Open-Shift [Back-End] Google Script: Write Update API [Fonrt-End] AJAX: Access |
[javascript, grid] How to create a spreadsheet -- adgrid (ref)
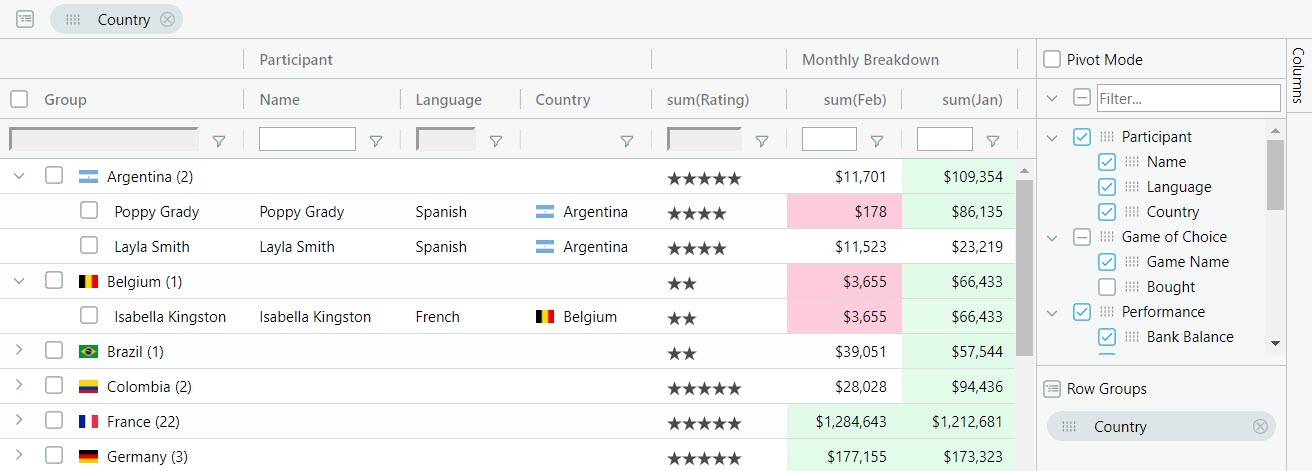
7. WebAPI
7.1. Console
[console, hex] How to print out a message in hex (ref)
let buf:Buffer = Buffer.from([1, 2, 3]); console.log('Data in Hex = ', buf.toString('hex') |
[console, raw2hex] How to convert the raw byte array to hex (ref)
function handleNotifications(event) { let value = event.target.value; let a = []; // Convert raw data bytes to hex values just for the sake of showing something. // In the "real" world, you'd use data.getUint8, data.getUint16 or even // TextDecoder to process raw data bytes. for (let i = 0; i < value.byteLength; i++) { a.push('0x' + ('00' + value.getUint8(i).toString(16)).slice(-2)); } log('> ' + a.join(' ')); }
|
7.2. Keyboard
keyboard event (view)
7.3. Touch
Touch UI Library
Sencha (view), very good
TouchSwipe (view)
JQueryMobile (view)
Gesture Library
W3C Touch Spec
Tutorial (view)
7.4. Timer
[timer, interval] How to use timer (view)
function main() { simInterval = setInterval( () => { console.log('Hello'); }, 500); } |
[node, timer, timeout] How to set timeout (ref)
// normal version function main() { setTimeout(); }
function my_next(strMessage) { console.log('my_next::', strMessage); }
// argument version function main() { setTimeout(my_next.bind(null, 'this is a argument)); }
function my_next(strMessage) { console.log('my_next::', strMessage); } |
[node, date, time] How to show year-month-day-hour-min-sec
Code const date = new Date(); const dateTimeFormat = new Intl.DateTimeFormat('en-US', { year: 'numeric', month: 'numeric', day: '2-digit', hour: '2-digit', minute: '2-digit', second: '2-digit'}) const [{ value: month },,{ value: day },,{ value: year },,{ value: hour },,{ value: minute },,{ value: second }] = dateTimeFormat.formatToParts(date )
console.log(`${year}-${month}-${day}-${hour}-${minute}-${second}`)
Output 2020-8-28-04-17-38
References https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Intl/DateTimeFormat/DateTimeFormat https://stackoverflow.com/questions/3552461/how-to-format-a-javascript-date
|
[date] How to get date information (view)
[timestamp] How to get timestamp (view)
7.5. WebBluetooth
[webbluetooth] The WebBluetooth browser compatibility (ref)
[webbluetooth, check] How to check if the browser supports the Web Bluetooth (view)
if (!navigator.bluetooth) { document.write("Web Bluetooth is not supported."); } |
7.6. WebAudio
[sheet] The WebAudio sheet (sheet)
[node, audio, format] How to get the wave format (ref)
var fs = require('fs'); var wav = require('wav');
strFullFilename = './ImperialMarch60_mono_signed_16bit_pcm_22k.wav'; var file = fs.createReadStream(strFullFilename); var reader = new wav.Reader(); // the "format" event gets emitted at the end of the WAVE header reader.on('format', function (format) { console.log('format = ', format) }); // pipe the WAVE file to the Reader instance file.pipe(reader);
|
[webaudio, source] How to create Sin audio buffer source for WebAudio to streaming play (view)
let source:AudioBufferSourceNode = this.__audioCtx.createBufferSource(); source.buffer = myAudioBuffer; // <<------- source.connect(this.__audioCtx.destination); source.start(this.__numStartAt);
|
[audio, seek] seek (view), (ref)
[audio] playback, m4a (view)
[audio, gain, change] How to change the audio gain dynamicly (view)
[audio, filter, bandpass] How to setup bandpass filter using webaudio (view)
[audio] audio2array, array2audio, playback, wavefile object (ref)
[audio, wavefile] How to read a wave file (ref)
const WaveFile = require('wavefile');
// read the audio file let buffer = fs.readFileSync(fullfilename_snd) let wav = new WaveFile(buffer); console.log('wav = ', wav) |
[audio, audio2sample) How to read the audio samples
Key
sample = wav.getSample(i)
const WaveFile = require('wavefile');
// read the audio file let buffer = fs.readFileSync(fullfilename_snd); let wav = new WaveFile(buffer); console.log('wav = ', wav);
// audio2buffer console.log('chunkSize = ', wav.data.chunkSize); console.log('bitsPerSample = ', wav.fmt.bitsPerSample) numOfSample = wav.data.chunkSize/wav.fmt.bitsPerSample console.log('numOfSample = ', numOfSample) for(i = 0; i < numOfSample; i++){ sample = wav.getSample(i) console.log(i, 'sample = ', sample) }
|
[audio, sample2audio] How to write a wave file from a sample array
console.log('chunkSize = ', wav.data.chunkSize); console.log('bitsPerSample = ', wav.fmt.bitsPerSample) numOfSample = Math.floor(wav.data.chunkSize*8/wav.fmt.bitsPerSample) console.log('numOfSample = ', numOfSample)
var int16SampleBuffer = new Int16Array(numOfSample);
for(i = 0; i < numOfSample; i++){ sample = wav.getSample(i) // console.log(i, 'sample = ', sample) int16SampleBuffer[i] = sample }
// buffer2wav let wav2 = new WaveFile(); wav2.fromScratch(1, 8000, '16', int16SampleBuffer); fs.writeFileSync('wav2.wav', wav2.toBuffer());
|
[webaudio, source, rxjs] How to create Sin audio buffer source for WebAudio to streaming play (view)
[webaudio, get data] How to get the mp3 data from remote URL (view)
[webaudio, algo, queue, cache] How to create a cache for storing the incoming variance length data (ref)
Q: 如果不要用 queue, 直接使用 Buffer. 不斷往後 concats, 避開切割的亂雜運算,是否能解決 glitch?
1. 加入資料 this.cache = Buffer.alloc(0)
this.cache = Buffer.concat([this.cache, buffer])
2. 取出資料: 用 slice(0, numbyteperframe] 從前面取資料
while (this.cache.length > 960) { const buffer = this.cache.slice(0, 960) // 取資料 this.cache = this.cache.slice(960) // 滑動 const samples = new Int16Array(new Uint8Array(buffer).buffer) this.onData({ bitsPerSample: 16, sampleRate: 48000, channelCount: 1, numberOfFrames: samples.length, type: 'data', samples }) } |
[webaudio, audioworklet] What is the AudioWorklet (ref)
[webaudio, audioworklet] How to create a white-noise source using Audio Worklet Node (view)
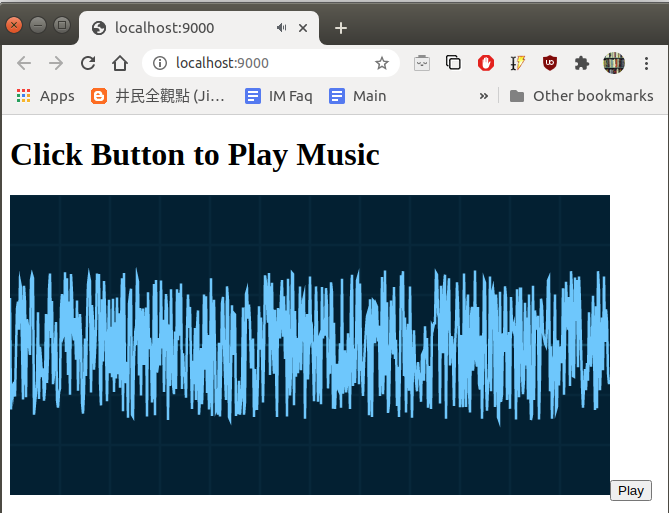
[webaudio, playback, webrtc] How to play a WebRTC stream with an audio element and add sound effect in real-time (view)
const source = context.createMediaStreamSource(remoteStream); source.connect(filter); filter.connect(gainNode); gainNode.connect(context.destination); |
[webaudio, render, canvas, Scope, javascript] How to visualize the web audio using scope using Scope with javascript** (view)
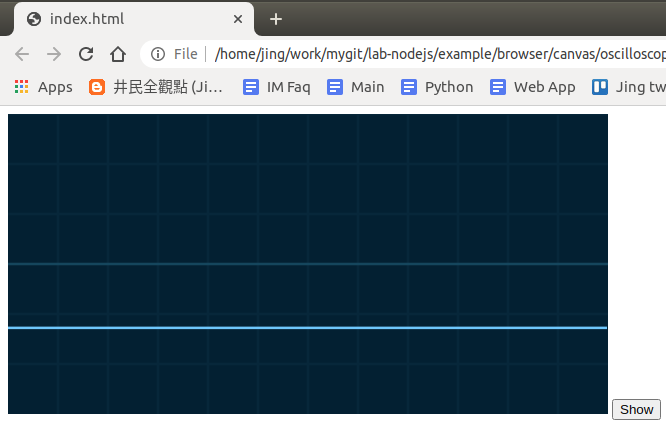
[webaudio, render, canvas, Scope, react] How to visualize the web audio using scope with React*** (view)
[webaudio, render, canvas, react] Audio visualisation with the Web Audio API and React (ref)
7.7. WebRTC
[webrtc, faq] The WebRTC FAQ (view)
[webrtc] How to implement a webrtc audio source to deliver WAV file (download)
rtcaudiosourcewavefile.js
let byteBuffer = fs.readFileSync(strFullFilename); let offset = 44; function next() { for (let i = 0; i < numberOfFrames; i++) { if (offset < byteBuffer.length - 2){ samples[i] = byteBuffer.readInt16LE(offset); }else{ //console.log('offset > byteBuffer.length - 2'); samples[i] = 0; offset = 0; } offset = offset + 2; // 16-bit = 2 bytes } //console.log('frame ', cntFrame, ' samples[0] = ', samples[0]); cntFrame++; source.onData(data); // eslint-disable-next-line scheduled = options.schedule(next, secondsPerSample*numberOfFrames*1000 ); }
let scheduled = options.schedule(next);
|
7.8. WebAssembly
[webAssembly, official] The official site (ref)
7.9. WebSerial
[webserial, faq] The webserial sheet (view)
8. Animation libraries
8.1. Spring
[spring, official, example] examples (ref)
[spirnt, tutorial] video (ref)
8.2. Famer
[framer, official] site (ref)
9. Graphic libraries
9.1. Survey
The Data Visualisation Catalogue (ref)
Open Source Plotting Libraries (primarily for React) (ref)
9.2. 3D libraries
[graphosaurus] Draw 3D point on screen (view) (ref)
[elegans] Draw 3D point on screen (ref)
[three.js] Draw 3D (ref)
[three.js] How to use three.js (view)
9.3. Image libraries
[canvas-js, official] The official site (ref)
[canvas-js, showImage] How to show a canvas on your browser (view)
[canvas-js, text, background] How to show a text on canvas with background (view)
[canvas-js, text, height] How to get the font height (view)
[canvas-js, rectangle] How to show a rectangle on your browser (view)
[canvas-js, clean] How to clean the canvas (view)
[canvas-js, savetofile] How to save a canvas image to a local file (view)
9.4. Map, CesiumJS
[cesiumjs, init] The first 3D app (view)
[cesiumjs, camera] How to setup the camera fly to the target (view)
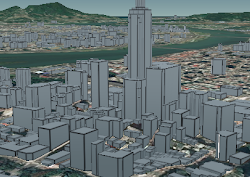
10. Chart libraries
10.1. comm
[chart, survey] Charting Library Survey (ref)
[chart, performance] Performance Test (ref)
Timechart (ref), uplot (ref) >> chart.js
10.2. High performance chart libraries
10.2.1. Dygraphs
[dygraphs, official] The official site (ref)
D3, speed: middle,
Javascript: ok, typescript: ok, React: ok
stream
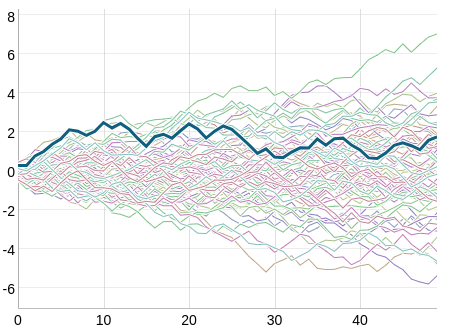
[dygraphs, performance] benchmarking for Plots with many points (ref), good enough
[dygraphs, play] A dynamic update sample on dygraphs in JSFiddle playground**** (view)(ref)
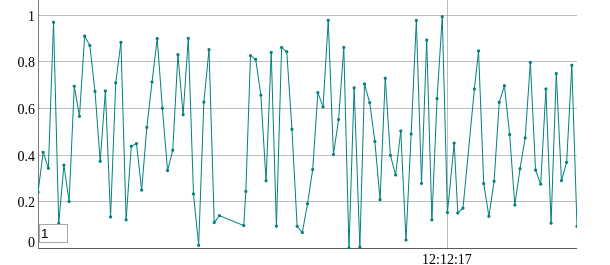
[dygraphs, react, real-time] Show live time-serial data using React, dygraphs, push/pull mode *** (view)
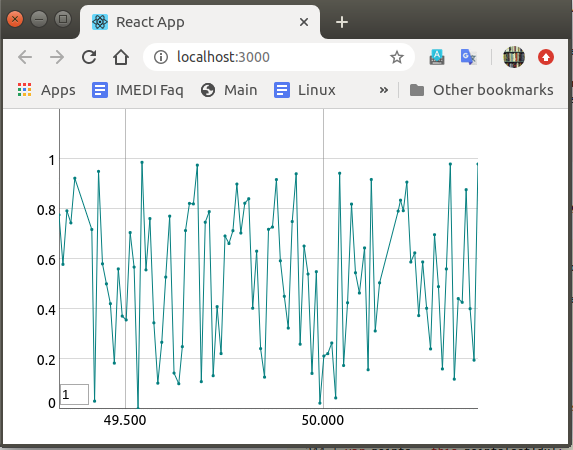
[react, function component, dygraph] How to show a live updated time-serial data using dygraphs in React function component (view)
10.2.2. TimeChart
[timechart, official] The official site (ref)
Webgl, very fast
Javascript: ok, React: ?
[timechart,, real-time] How to draw a live serial data in real-time (ref)
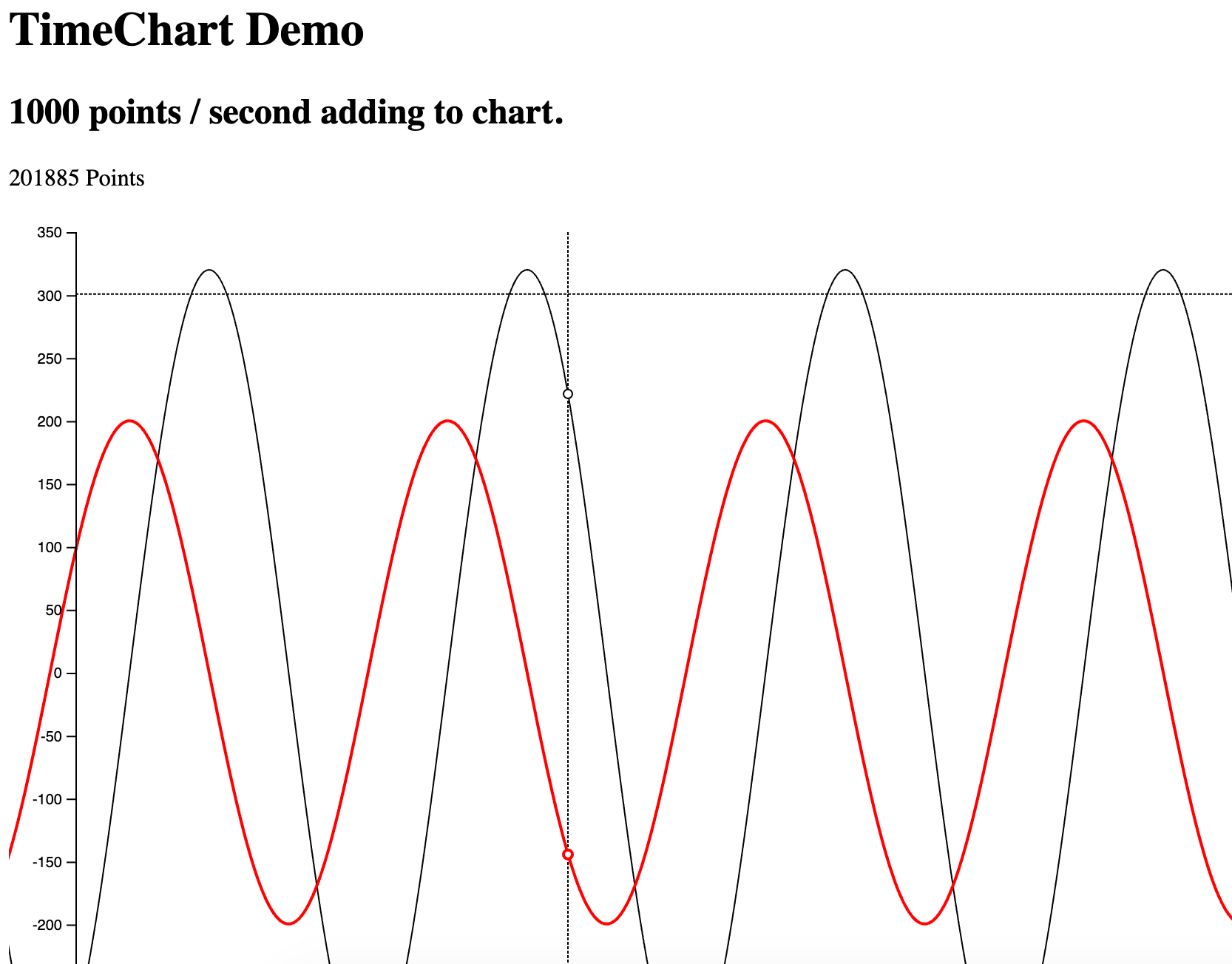
10.2.3. uPlot
[uplot, official] The official site (ref)
Webgl, very fast
Javascript: ok, React: yes
[uplot, real-time] How to draw a live serial data in real-time (ref)
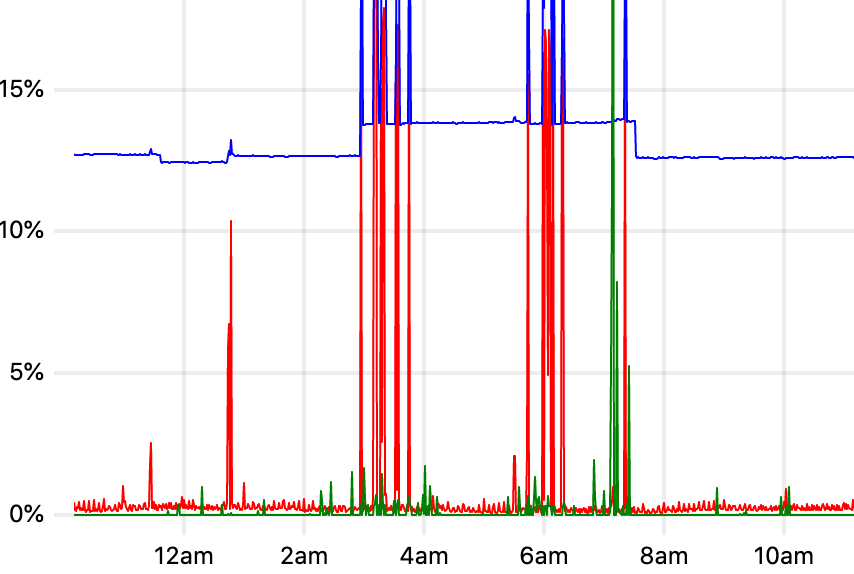
[uplot, react] a react example (ref)
[uplot, react, wrap] The react wrap (ref), (class vs. function ex)
[uplot, react, function] How to use uplot (github)
10.2.4. Webgl-plot
[webgl-plot, official] The official site (ref)
Webgl, very fast, width only 1
Javascript: ok, React: ?
[webgl-plot, real-time] How to draw a live serial data in real-time ** (ref)
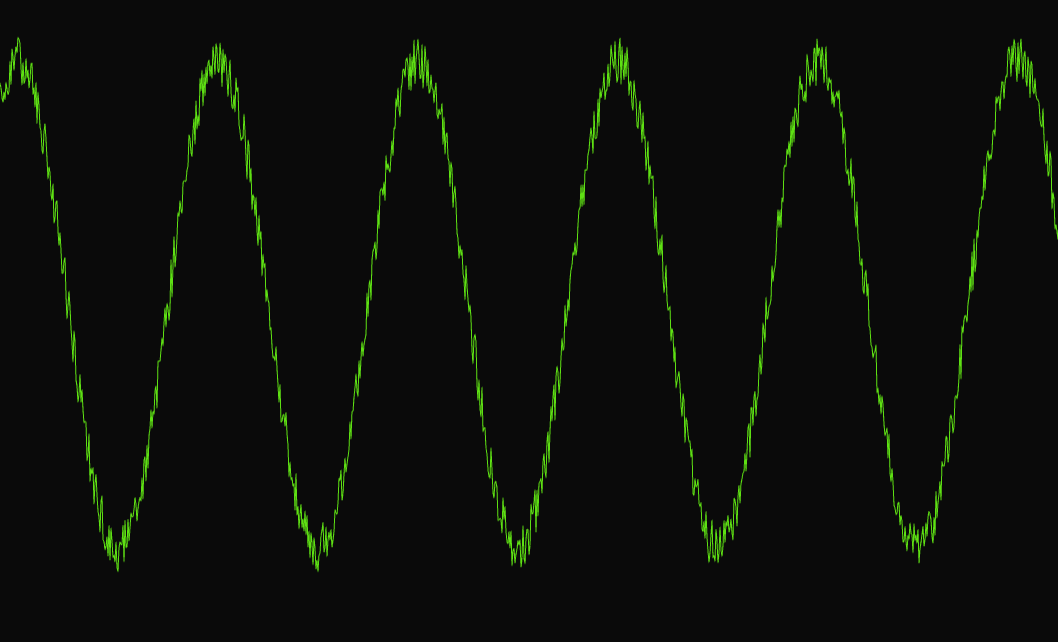
10.2.5. [canvas] d3
[d3, official] The official site, d3 (ref)
[d3, basic shape] Basic shape (ref)
[d3, circle] How to draw a circle (view), (js)
[d3, circle] How to draw 3 circle using data binding (view)
[d3, curve] (ref), (curve example)
[d3, real-time, d3] How to draw a live serail data in real-time (ref)
[d3, real-time, rickshaw] The rickshaw (ref), start: 6.5k
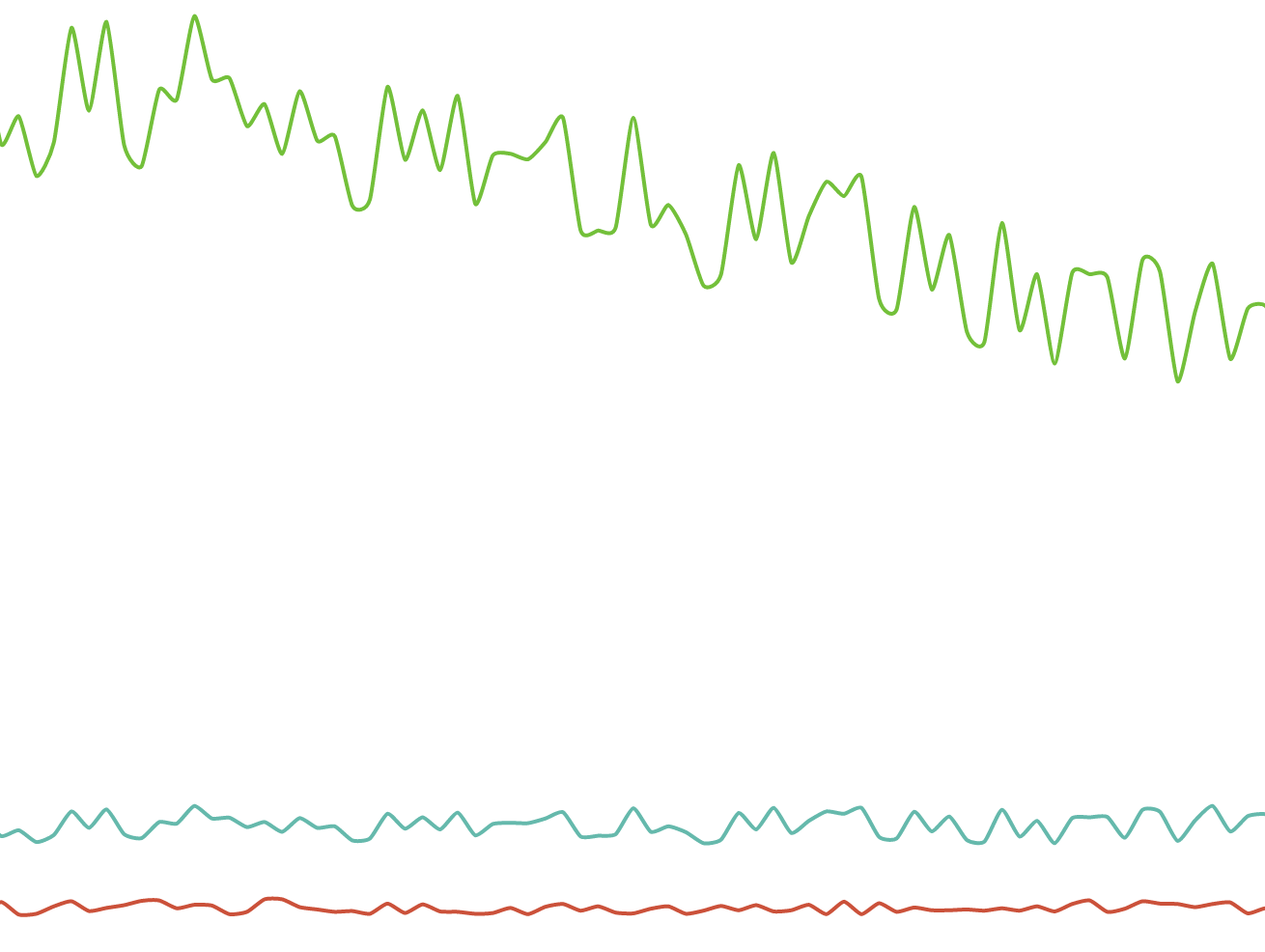
10.2.6. [canvas] Wavesurfer
1. [time-line] official (ref)
10.2.7. Livechart
[livechart, official] The official site (ref)
10.2.8. Chart.js
10.2.8.1. Javascript (chart.js only)
[chartjs, official] The official site (ref)
缺點: 速度太慢
[chartjs, javascript, line] How to use chartjs (view)
[node, chartjs] How to write a node code to use chartjs for charting (ref)
[chartjs, javascript, line, live] How to show a live line chart using pure javascript (view)***, 最簡單, 所有可執行的範例,
(how to use typescript to implement this?, 非同步測試?)
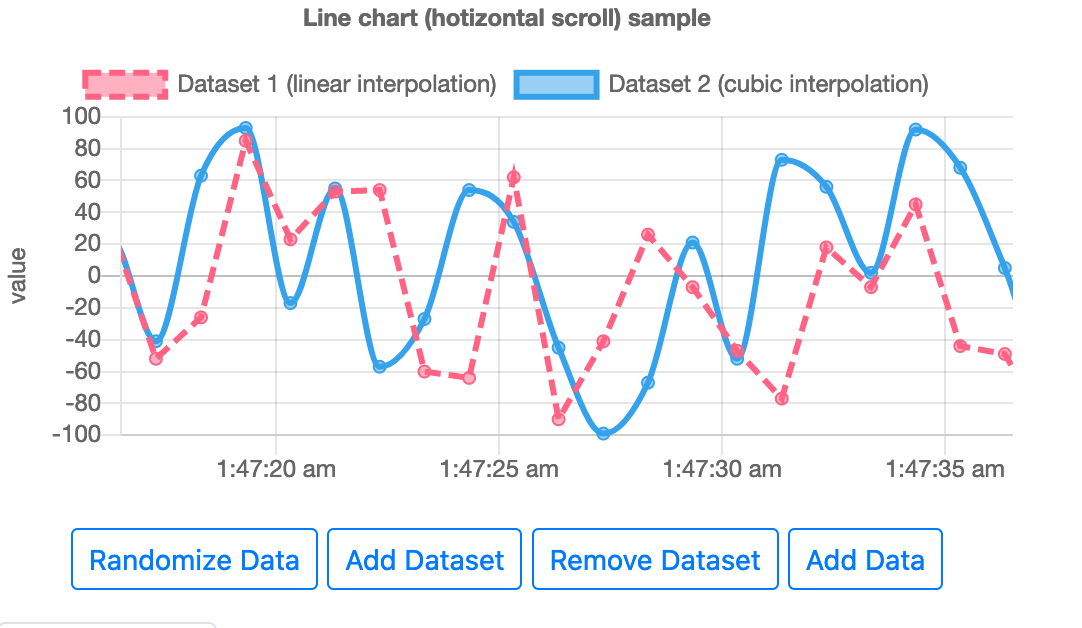
[chartjs, interact, pure] How to draw a live bar chart in real-time (ref) (github)
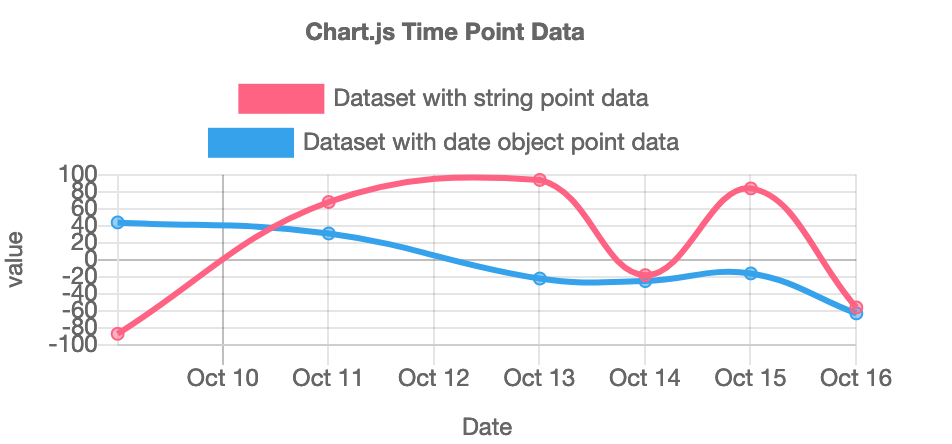
10.2.8.2. Typescript + React
[chartjs. react, typescript] How to show a static line chart with React (view)
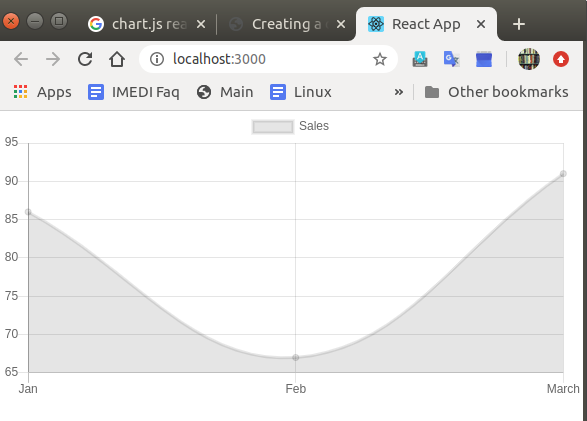
10.2.8.3. react-chartjs-2
[react-chartjs-2, real-time, ] How to draw a live line chart in real-time (view)
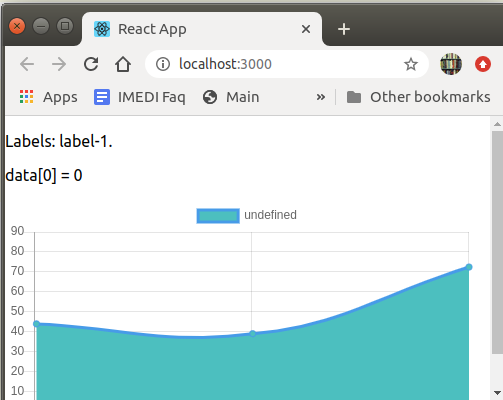
10.2.8.4. chartjs-plug-stream
[chartjs-plug-streaming, data feed] The Data feed models (ref)
[chartjs-plug-streaming, javascript] Show live time-serial data using pure javascript (html 版本順暢)
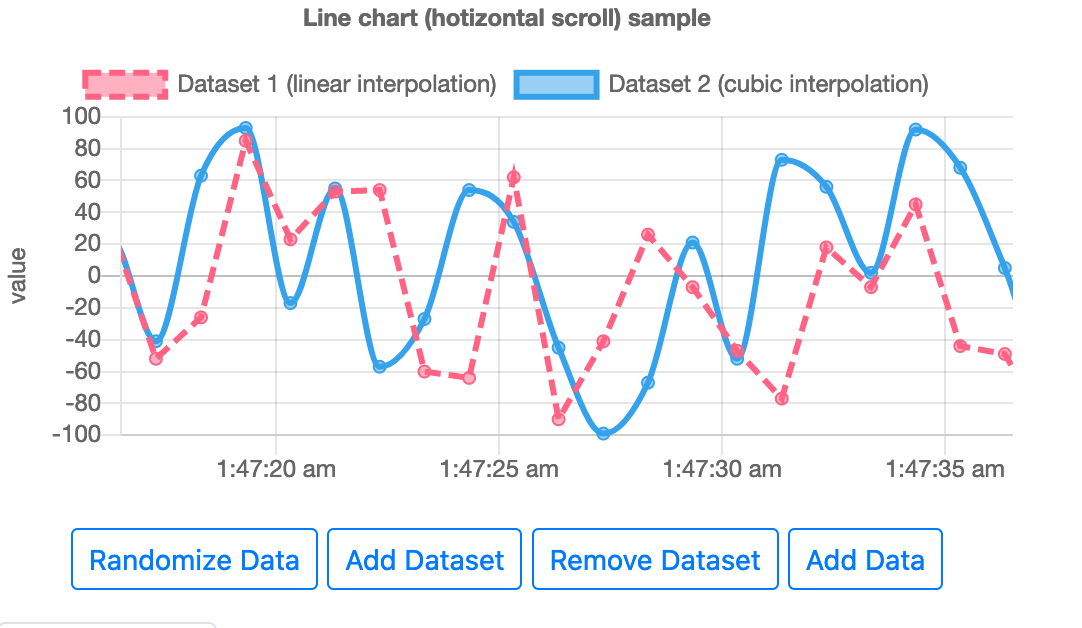
[chartjs-plug-streaming, react] Show live time-serial data using React*** (view react 版會頓一下, 奇怪)
React 版卡頓似乎跟 char.js 版本有關. solution yarn add chart.js@2.8.0 (比較順) yarn add chart.js@2.7.3 (比較順) |
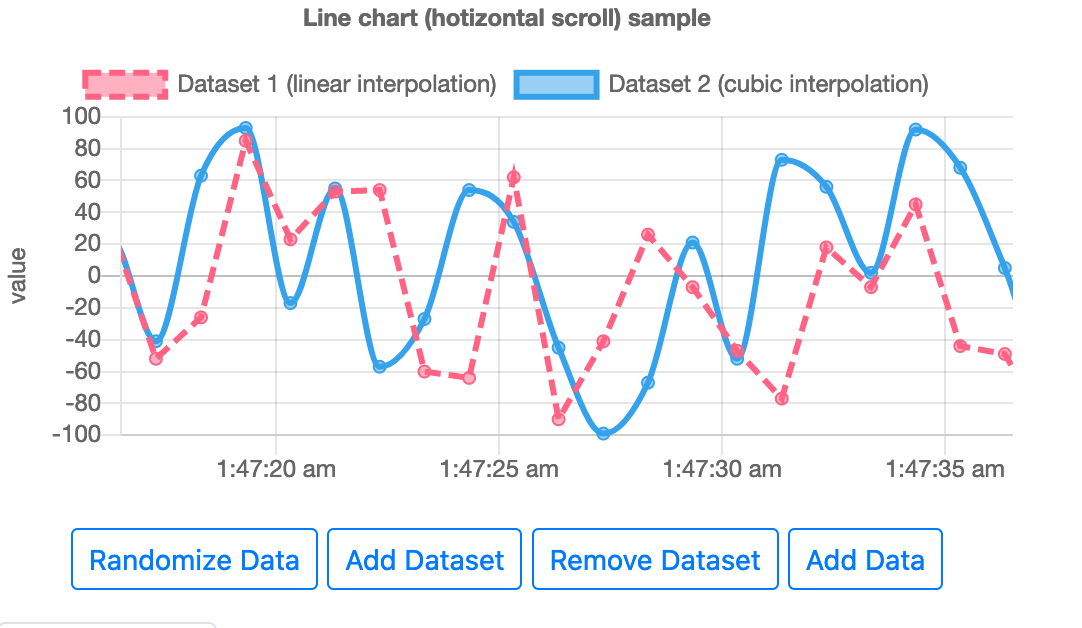
[chartjs-plug-streaming, chart] How to get the chart object from chart.js react object (ref)(official, react)
<Line data={this.state} height={5} width={20} ref = {(reference) => this.reference = reference} />
|
[chartjs-plug-streaming, react] Show live time-serial data using React with Push Model (Listening Based)*** (view)
Key:
class App extends Component { private myRefLine:any = null; // for Line chart obj ...
componentDidMount(){ ... if (this.myRefLine == null) return;
let myChart:any = this.myRefLine.chartInstance; myChart.data.datasets[0].data.push({ x: Date.now(), y: Math.floor(Math.random() * 100) }); // update chart datasets keeping the current animation myChart.update({ preservation: true }); ... }
render() { return ( <Line data={this.dictDataCfg} options={this.dictOptions} ref = {(ref) => this.myRefLine = ref} /> ); } |
|
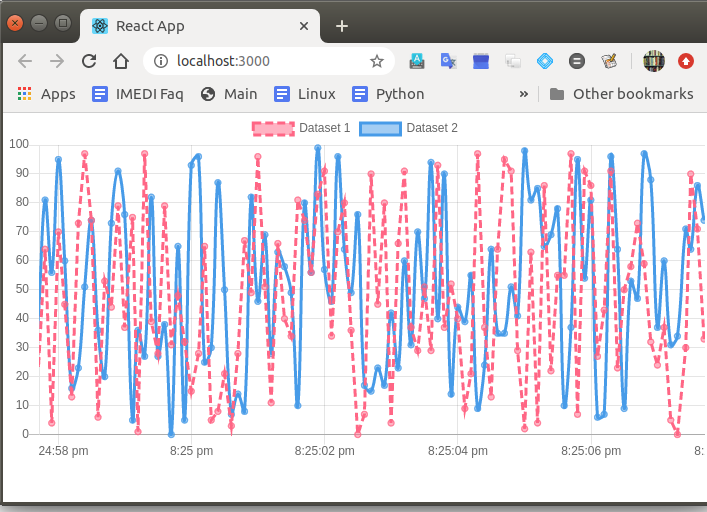
10.2.9. Recharts
[recharts, official] The examples (ref)
[recharts, real-time] How to draw a live bar chart in real-time (ref) (line)
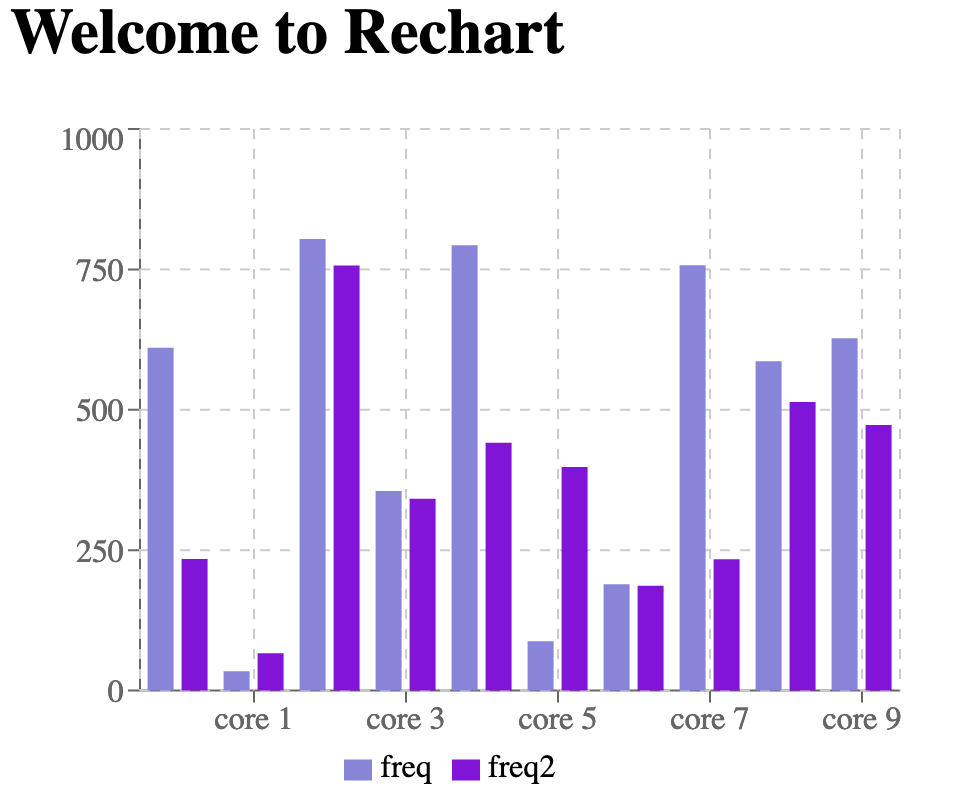
10.2.10. Others
[camera] communicate with the network camera (ref)
[animation, physical] CSS Animation (ref)
[chart, rea-time, epoch] (ref)